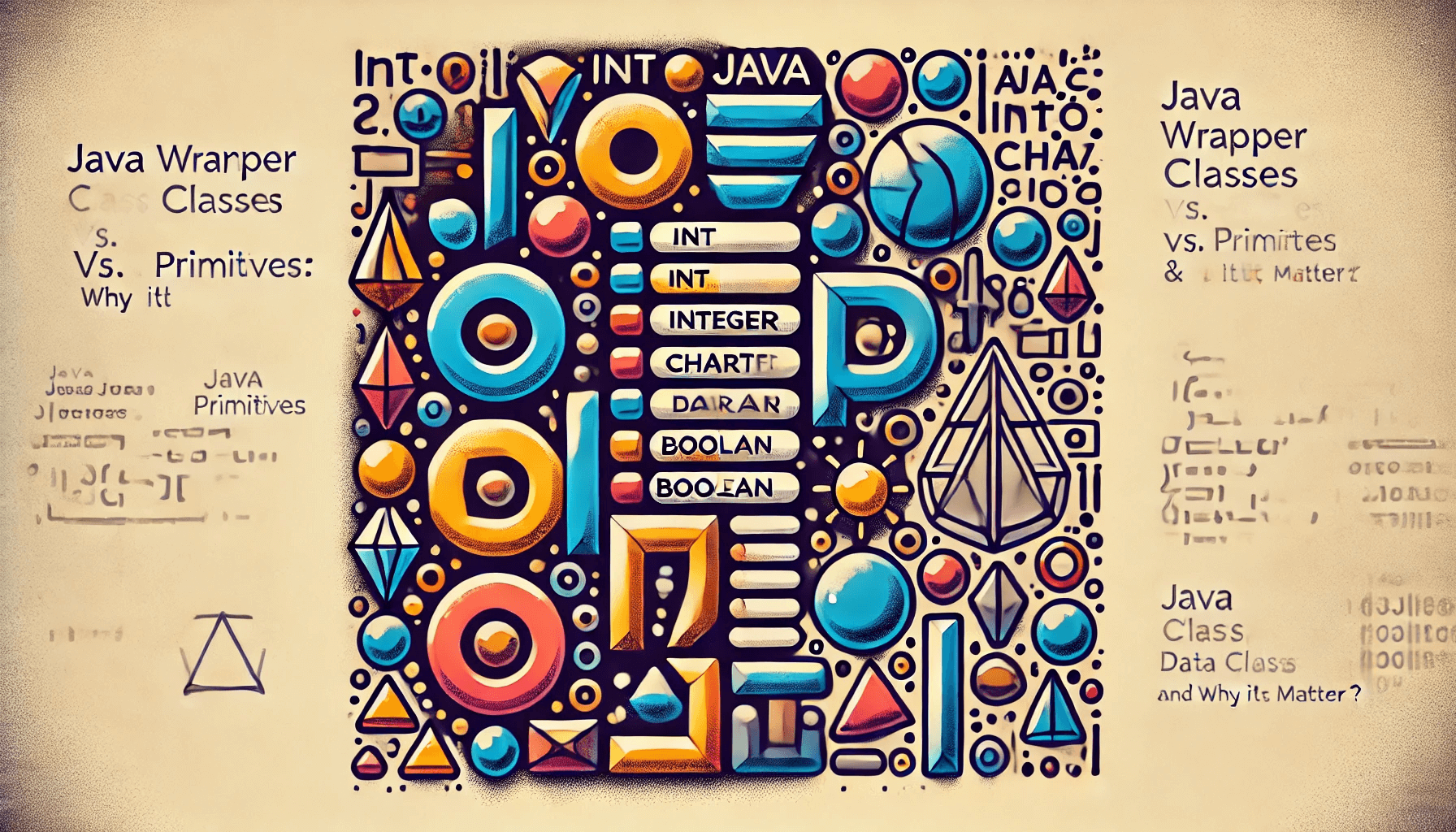
Java Wrapper Classes vs. Primitives: What's the Difference and Why Does It Matter?
My colleague asked me: “Why we don’t use primitives more? It is faster!”.
I didn’t really have a good answer at the time so this motivated me to write this article :)
Introduction
Working with Java often involves juggling two types of data representations: primitive types (such as int
,
double
, boolean
, etc.) and their wrapper class counterparts (like Integer
, Double
, Boolean
, etc.). On the
surface, they may look and function similarly, but under the hood, they behave quite differently. In this post, we’ll
explore the reasons behind having two categories of types in Java, how they differ, and scenarios where you’d want to
use one over the other.
What Are Primitives in Java?
Primitives in Java are basic data types that represent raw values. They’re not objects; they’re simply stored in memory locations that hold the value directly. Java defines the following eight primitive types:
- byte – 8-bit signed integer
- short – 16-bit signed integer
- int – 32-bit signed integer
- long – 64-bit signed integer
- float – 32-bit floating-point
- double – 64-bit floating-point
- char – 16-bit Unicode character
- boolean – true/false value
Key Characteristics of Primitives:
- Fast and lightweight, as they store only the value
- Not part of the object hierarchy, meaning they can’t have methods or be used with generics directly
- Have a fixed size, making them efficient for numeric and boolean operations
- Default values:
0
for numeric typesfalse
forboolean
\u0000
forchar
Example usage:
int count = 10;
double price = 19.99;
boolean isValid = true;
What Are Wrapper Classes in Java?
Wrapper classes are object representations of the primitive types. Each primitive has a corresponding wrapper class:
Byte
Short
Integer
Long
Float
Double
Character
Boolean
Key Characteristics of Wrapper Classes:
- They are full-fledged objects stored on the heap
- Offer utility methods for parsing, converting, or manipulating values
- Allow usage of
null
, which can be advantageous or problematic - Require more memory and have more overhead than primitives
Memory Layout Example:
Primitive int (4 bytes):
[0000 0000 0000 0000 0000 0000 0000 0000]
|<------------ 4 bytes (32 bits) ---------->|
- An int is stored directly in memory (usually on the stack)
- Takes exactly 4 bytes (32 bits)
- Can represent values from -2³¹ to 2³¹-1
- Direct memory access makes operations very fast
- No overhead - every bit is used for the actual value
Integer object (16 bytes minimum):
[ Object Header ][Mark Word][Klass Ptr][ Value ]
|<--- 8 bytes --->||<-- 4 bytes -->||<- 4 bytes ->|
|<-------------------- 16 bytes total -------------->|
Object Header (12 bytes total):
-
Mark Word (8 bytes):
- Contains information used by the JVM
- Includes: hash code, garbage collection state, locking state
- Used for synchronization and memory management
-
Klass Pointer (4 bytes):
- Points to the class metadata
- Tells JVM this is an Integer object
- Enables object-oriented features
Actual Value (4 bytes):
-
The same 4 bytes as the primitive int
-
Stored on the heap instead of the stack
-
Referenced through the object
Autoboxing and Unboxing
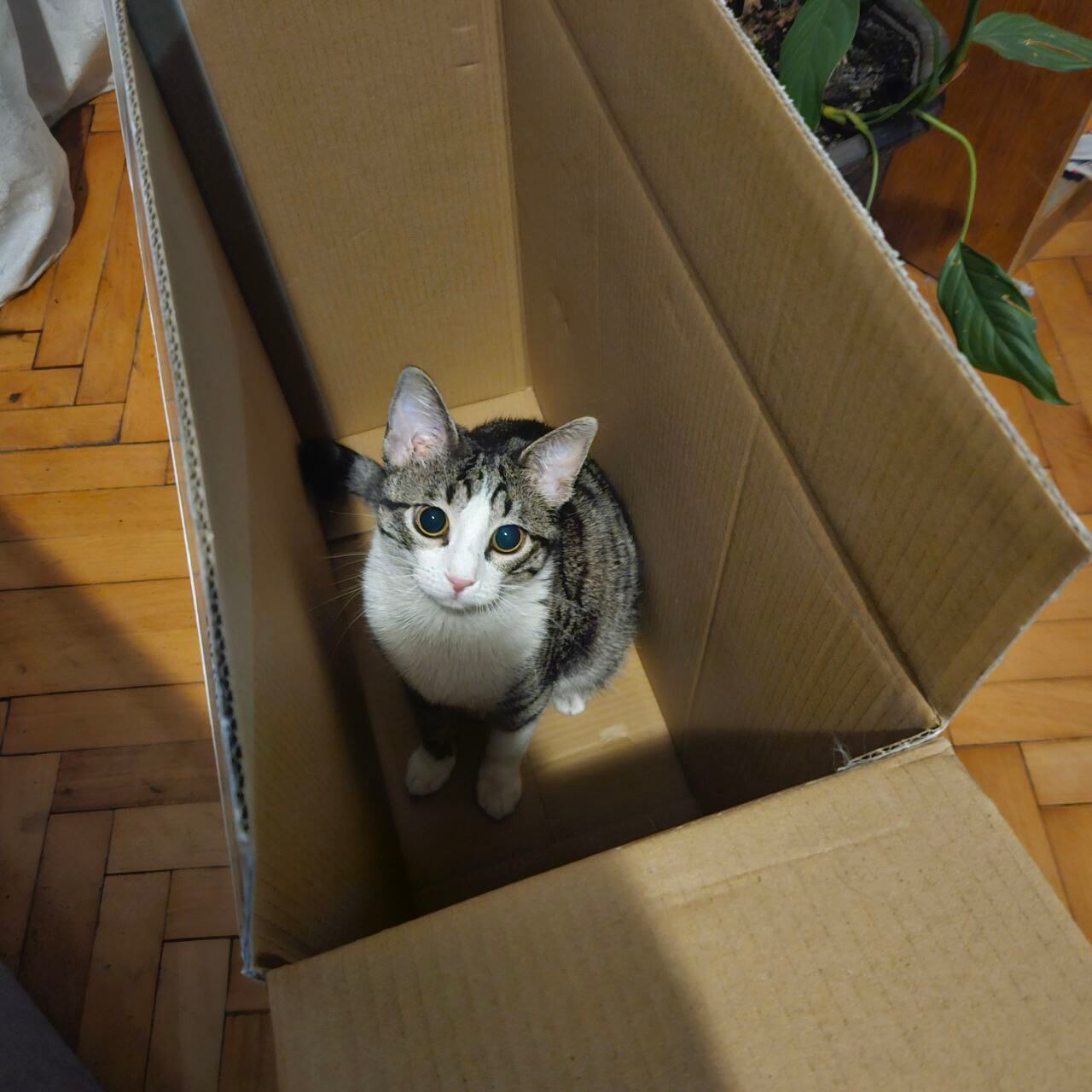
One of the key features that bridges the gap between primitives and wrapper classes is autoboxing and unboxing.
-
Autoboxing: The automatic conversion of a primitive type to its corresponding wrapper class.
Example:int num = 5; Integer obj = num; // Autoboxing (int -> Integer)
-
Unboxing: The automatic conversion of a wrapper class object to its corresponding primitive type.
Example:Integer obj = 5; int num = obj; // Unboxing (Integer -> int)
While autoboxing and unboxing make the language more convenient, they can also introduce performance pitfalls and *
NullPointerExceptions* if not used carefully (e.g., unboxing a null
Integer
).
Key Differences
-
Performance:
Let’s look at a concrete example:// Benchmark example long start = System.nanoTime(); for (int i = 0; i < 10_000_000; i++) { int primitive = i + 1; // Primitive operation } long primitiveTime = System.nanoTime() - start; start = System.nanoTime(); for (Integer i = 0; i < 10_000_000; i++) { Integer wrapper = i + 1; // Boxing/unboxing operation } long wrapperTime = System.nanoTime() - start;
Typical results show wrapper operations are 15-20x slower than primitives.
-
Memory Usage:
- Primitives consume fixed memory (e.g., int = 4 bytes)
- Wrappers have object overhead (e.g., Integer = 16 bytes minimum) Memory layout breakdown for Integer:
- 8 bytes: Mark Word (hash code, GC state, locking)
- 4 bytes: Klass Pointer (class metadata)
- 4 bytes: Actual int value
-
Default Values & Nullability:
- Primitives cannot be
null
; they have default values - Wrappers can be assigned
null
- Primitives cannot be
-
Object Methods:
- Wrappers have built-in utility methods
- Primitives do not have methods
-
Generics Compatibility:
- Primitives cannot be used in generics
- Wrappers can be used as type parameters
Real-World Applications
When to Use Primitives:
- Game Development
public class GamePhysics {
private double positionX;
private double positionY;
public void updatePosition(double deltaTime) {
positionX += velocityX * deltaTime;
positionY += velocityY * deltaTime;
}
}
- Real-time Processing
public class SignalProcessor {
public double[] processSignal(double[] rawData) {
double[] processed = new double[rawData.length];
for (int i = 0; i < rawData.length; i++) {
processed[i] = rawData[i] * 1.5;
}
return processed;
}
}
When to Use Wrappers:
- Database Entities
@Entity
public class User {
@Id
private Long id;
private Integer age;
private Boolean isActive;
}
- API Responses
public class ProductDTO {
private Integer productId;
private Double price;
private Boolean isAvailable;
}
Modern Java Features
Optional and Wrapper Classes
public class UserService {
public Optional<Integer> getUserAge(String userId) {
User user = findUser(userId);
return Optional.ofNullable(user.getAge());
}
}
Stream API Considerations
// Primitive stream (efficient)
IntStream.range(0, 1000000)
.sum();
// Wrapper stream (less efficient)
Stream.iterate(0, i -> i + 1)
.limit(1000000)
.mapToInt(Integer::intValue)
.sum();
Common Pitfalls and Best Practices
- Accidental Null Unboxing
Integer countObj = null;
// This will throw NullPointerException
int count = countObj;
// Better approach
int safeCount = (countObj != null) ? countObj : 0;
- Performance in Loops
// Bad practice (boxing/unboxing overhead)
List<Integer> numbers = new ArrayList<>();
for (Integer i = 0; i < 1000000; i++) {
numbers.add(i);
}
// Better approach
IntStream.range(0, 1000000)
.boxed()
.collect(Collectors.toList());
- Comparing Values
Integer x = 127;
Integer y = 127;
System.out.println(x == y); // true (due to caching)
Integer a = 128;
Integer b = 128;
System.out.println(a == b); // false
System.out.println(a.equals(b)); // true (correct way)
- Boolean Comparisons
Boolean flag1 = Boolean.TRUE;
Boolean flag2 = Boolean.TRUE;
// Use equals() instead of ==
boolean result = flag1.equals(flag2);
Decision Guidelines
When choosing between primitives and wrappers, consider:
-
Do you need null values?
- Yes → Use wrapper
- No → Consider primitive
-
Is performance critical?
- Yes → Use primitive
- No → Either is fine
-
Need collections or generics?
- Yes → Use wrapper
- No → Consider primitive
Conclusion
Understanding the trade-offs between primitives and wrapper classes is crucial for writing efficient Java code. Use primitives for performance-critical operations and when null values aren’t needed. Use wrappers when working with collections, frameworks, or when null values are part of your domain model.
Additional Resources
- Official Java Documentation on Primitive Data Types
- Autoboxing/Unboxing Documentation
- Effective Java by Joshua Bloch
- Java Performance: The Definitive Guide
Happy coding!